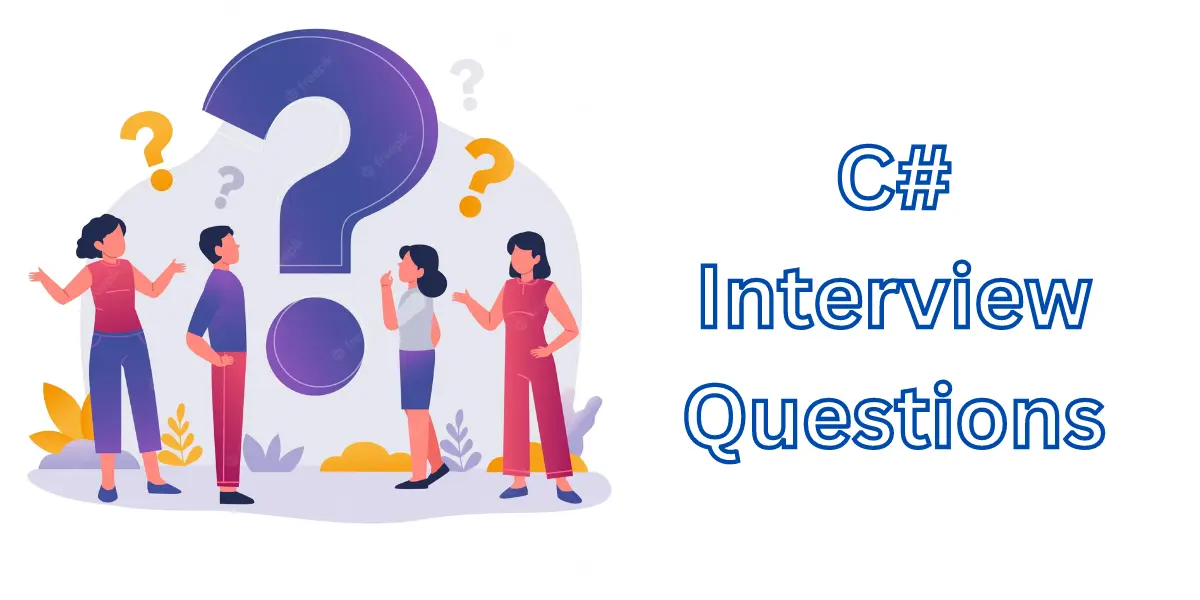
C# Interview Questions
What is C#?
C# (pronounced as “C sharp”) is a modern, object-oriented programming language developed by Microsoft as part of its .NET initiative. C# was designed to be simple, powerful, and versatile, with a focus on productivity and ease of use. It is primarily used for developing desktop, web, and mobile applications on the Microsoft platform, including Windows, Windows Phone, and Azure cloud services.
Key Features and characteristics of C# include:
- Object-Oriented: C# follows the principles of object-oriented programming (OOP), allowing developers to define classes, create objects, and implement concepts like encapsulation, inheritance, and polymorphism.
- Type-Safe and Strongly Typed: C# is a type-safe language, which means it enforces type checking during compilation to catch type-related errors early. It also has strong type checking, requiring explicit declaration of variable types.
- Garbage-Collection: C# uses automatic memory management through garbage collection, freeing developers from manual memory management tasks and reducing the risk of memory leaks.
- Asynchronous Programming: C# has robust support for asynchronous programming using async/await, allowing developers to write responsive and efficient applications.
- Unified Type System: C# employs a unified type system that includes both value types (structs) and reference types (classes), providing flexibility and performance optimization.
- LINQ (Language-Integrated Query): C# supports LINQ, a powerful feature that allows developers to perform data queries directly within the language, making it easier to work with data collections.
- Extensive Standard Library: C# comes with a rich standard library, the .NET Framework, which provides pre-built classes and APIs for various tasks like file I/O, networking, cryptography, database access, and more.
- Cross-Language Integration: C# is designed to interoperate with other .NET languages, enabling developers to use libraries and components written in different languages within the same application.
- Cross-Platform Development: With the advent of .NET Core and the .NET 5+ platform, C# has become more cross-platform. It allows developers to build applications for Windows, macOS, and Linux.
C# is widely used for a variety of software development purposes, including creating Windows desktop applications using Windows Forms or WPF, developing web applications using ASP.NET, building mobile apps with Xamarin, and creating cloud-based solutions on Microsoft Azure.
C# has gained popularity due to its ease of use, powerful features, and tight integration with the Microsoft ecosystem, making it a popular choice for developers targeting Microsoft platforms and technologies.
What is the difference between static, public, and void?
static
, public
, and void
are three distinct keywords used in the context of methods (functions) in programming languages like Java and C#. Each of these keywords serves a specific purpose and modifies the behavior of a method in different ways.
- static:
static
is a keyword used to define a method as a class-level method, also known as a static method.- A static method belongs to the class itself, not to any specific instance (object) of the class.
- Static methods can be called using the class name directly, without creating an instance of the class.
- They are commonly used for utility functions, helper methods, and operations that do not depend on the state of any particular object.
Example of a static method:
public class MathUtils {
public static int add(int a, int b) {
return a + b;
}
}
// Calling the static method:
int sum = MathUtils.add(5, 3);
- public:
public
is an access specifier used to define the visibility or accessibility of a method.- A
public
method can be accessed from any other class, regardless of whether it’s in the same package or a different package. - It provides the widest level of visibility for methods and is often used for methods that need to be accessible from multiple parts of the application.
Example of a public method:
public class MyClass {
public void printMessage() {
System.out.println("Hello, world!");
}
}
// Calling the public method:
MyClass obj = new MyClass();
obj.printMessage();
- void:
void
is a return type used to indicate that a method does not return any value.- When a method is declared with a
void
return type, it means the method does not produce a result, and it will not have areturn
statement that returns a value. void
is used for methods that perform operations or tasks but do not need to return a value.
Example of a method with a void return type:
public class Printer {
public void print(String message) {
System.out.println(message);
}
}
// Calling the void method:
Printer printer = new Printer();
printer.print("Hello, world!");
In summary, static
, public
, and void
are keywords used to define different aspects of methods:
static
: Defines a class-level method that is not associated with any instance of the class.public
: Specifies that the method can be accessed from any other class.void
: Indicates that the method does not return any value.
What is the benefit of ‘using’ statement in C#?
In C#, the using
statement is used primarily for two purposes, each providing distinct benefits:
- Resource Management and Disposal:
The primary benefit of theusing
statement is to manage resources that implement theIDisposable
interface. TheIDisposable
interface is used to release unmanaged resources or perform cleanup operations before an object goes out of scope. Resources like file handles, database connections, network sockets, and other system-level resources should be properly managed to avoid resource leaks and ensure efficient memory usage. When you use theusing
statement, it ensures that theDispose()
method of the object is called automatically when the code block inside theusing
statement is exited, either through normal execution or an exception. This way, resources are properly released, even if an exception is thrown. Example of usingusing
with a resource that implementsIDisposable
:
using (var fileStream = new FileStream("example.txt", FileMode.Open))
{
// Perform operations using the file stream
} // The file stream is automatically disposed here
In this example, the FileStream
object is automatically disposed when it goes out of the using
block, even if an exception occurs inside the block.
- Alias for Namespaces:
Another use of theusing
statement is to create aliases for namespaces, especially when dealing with types that have the same name in different namespaces. It allows you to avoid naming conflicts and provides a more concise and readable code. Example of usingusing
for namespace alias:
using System.IO;
using AnotherNamespace = MyCompany.OtherProject.Utilities;
// Now, you can use classes from both System.IO and MyCompany.OtherProject.Utilities namespaces
In this example, System.IO
is used as usual, but MyCompany.OtherProject.Utilities
is aliased as AnotherNamespace
to avoid naming conflicts.
Using the using
statement for resource management helps ensure that resources are released in a timely manner, preventing potential memory leaks and improving the overall performance and reliability of the application. Additionally, using using
to create aliases for namespaces enhances code readability and makes it easier to work with types from different namespaces that might have similar names.
What is serialization?
Serialization is the process of converting an object into a format that can be easily stored, transmitted, or reconstructed later. In other words, it is the process of converting an object’s state (its data) into a byte stream or some other portable format, which can be saved to a file, sent over a network, or persisted in a database.
The primary purposes of serialization are:
- Persistence: By serializing objects, their state can be saved to a file or database and later retrieved to restore the object’s state when needed. This is often used in applications that need to save user settings, game progress, or any other application state that should persist across sessions.
- Inter-Process Communication (IPC): Serialization is used when passing objects between different processes or over a network. When communicating between different systems or platforms, objects need to be converted into a format that can be easily transmitted and reconstructed on the other side.
- Distributed Systems: In distributed systems, different components may need to exchange data with each other. Serialization allows these components to send and receive complex objects in a format that can be understood and reconstructed by different parts of the system.
In many programming languages, including Java and C#, objects that need to be serialized must implement a special interface or inherit from a specific base class that marks them as serializable. In Java, this interface is java.io.Serializable
, and in C#, it is System.Runtime.Serialization.ISerializable
. Implementing this interface enables the language runtime to understand how to convert the object’s state into a serialized form and vice versa.
Here’s a simplified example of serialization in C#:
using System;
using System.IO;
using System.Runtime.Serialization;
using System.Runtime.Serialization.Formatters.Binary;
[Serializable]
public class Person : ISerializable
{
public string Name { get; set; }
public int Age { get; set; }
public Person() { }
public Person(SerializationInfo info, StreamingContext context)
{
Name = info.GetString("Name");
Age = info.GetInt32("Age");
}
public void GetObjectData(SerializationInfo info, StreamingContext context)
{
info.AddValue("Name", Name);
info.AddValue("Age", Age);
}
}
class Program
{
static void Main()
{
Person person = new Person { Name = "Alice", Age = 30 };
// Serialize the person object to a file
using (FileStream fileStream = new FileStream("person.dat", FileMode.Create))
{
BinaryFormatter formatter = new BinaryFormatter();
formatter.Serialize(fileStream, person);
}
// Deserialize the person object from the file
using (FileStream fileStream = new FileStream("person.dat", FileMode.Open))
{
BinaryFormatter formatter = new BinaryFormatter();
Person deserializedPerson = (Person)formatter.Deserialize(fileStream);
Console.WriteLine("Name: " + deserializedPerson.Name);
Console.WriteLine("Age: " + deserializedPerson.Age);
}
}
}
In this example, the Person
class is marked as [Serializable]
and implements ISerializable
. The object is serialized to a file using the BinaryFormatter
, and then it is deserialized back to an object. When deserialized, the object is restored to its original state, and we can access its properties as before.
List the different types of comments in C#
In C#, there are three main types of comments that you can use to add explanatory notes, documentation, or remarks to your code. These comments are ignored by the compiler and do not affect the execution of the program. The three types of comments in C# are:
- Single-line Comments:
Single-line comments start with two forward slashes (//
). Anything written after the//
on the same line is considered a comment and will not be executed by the compiler. Single-line comments are useful for adding short explanations or comments to specific lines of code.
Example of a single-line comment:
// This is a single-line comment in C#
int age = 30; // This variable stores the person's age
- Multi-line Comments (Block Comments):
Multi-line comments start with/*
and end with*/
. Everything between these delimiters is considered a comment and will not be executed. Multi-line comments are used when you want to add longer explanations or multiple lines of comments.
Example of a multi-line comment:
/*
This is a multi-line comment in C#.
It can span multiple lines without using // at the beginning of each line.
*/
int result = 0;
/* The following code calculates the sum of two numbers */
result = 5 + 3;
- XML Documentation Comments:
XML documentation comments start with three forward slashes followed by a double asterisk (///
). These comments are specifically used to generate documentation for your code using tools like Visual Studio’s IntelliSense and documentation generators like Sandcastle or Doxygen.
Example of an XML documentation comment:
/// <summary>
/// This method adds two integers and returns the result.
/// </summary>
/// <param name="a">The first integer.</param>
/// <param name="b">The second integer.</param>
/// <returns>The sum of a and b.</returns>
public int Add(int a, int b)
{
return a + b;
}
XML documentation comments are essential for providing clear and helpful documentation to other developers who use your code or library. They describe the purpose of the method, its parameters, and the return value, making it easier for others to understand and use your code effectively.
Remember to use comments appropriately and consistently to make your code more readable, maintainable, and understandable by yourself and other developers.