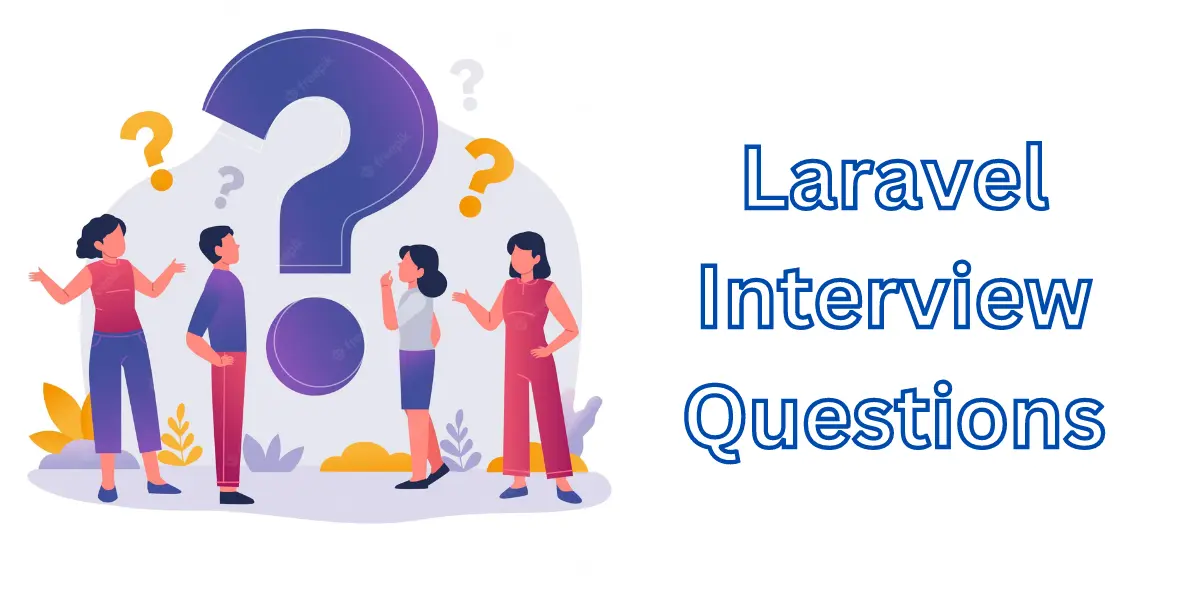
Laravel Interview Questions
What is Laravel?
Laravel is an open-source PHP web framework used for building web applications and APIs. It was created by Taylor Otwell and released in 2011. Laravel follows the Model-View-Controller (MVC) architectural pattern, which provides a structured way to organize code and separates application logic from presentation.
Key features of Laravel include:
- Eloquent ORM: Laravel provides an elegant and intuitive Active Record implementation called Eloquent. It allows developers to interact with the database using PHP syntax instead of writing raw SQL queries.
- Artisan Console: Laravel comes with a powerful command-line interface called Artisan, which provides various useful commands for tasks such as generating code, migrating databases, and running unit tests.
- Blade Templating Engine: Laravel uses the Blade templating engine, which enables developers to write clean and efficient templates with dynamic content while maintaining a clear separation between PHP code and HTML.
- Routing System: Laravel offers a robust routing system that makes it easy to define application URLs and map them to corresponding controller actions.
- Middleware: Middleware allows you to filter HTTP requests entering your application. It provides a convenient mechanism for adding layers of logic before the request is handled by the application.
- Authentication and Authorization: Laravel makes it simple to implement user authentication and access control features, including user registration, login, and managing user permissions.
- Security: Laravel comes with built-in security features such as CSRF (Cross-Site Request Forgery) protection, secure hashing, and encryption mechanisms.
- Testing: Laravel supports unit testing out of the box with PHPUnit. This makes it easier to ensure that new changes or features do not introduce unexpected bugs.
- Database Migrations and Seeding: Laravel provides a migration system for versioning the database structure and seeding it with test data, making database setup and maintenance easier.
Laravel has gained immense popularity within the PHP community due to its simplicity, expressive syntax, extensive documentation, and active community support. It has become one of the go-to choices for PHP developers when building modern web applications and APIs.
What is the latest Laravel version?
Laravel 9 was released on February 8, 2022. Laravel 10 was released on February 14, 2023.
Define composer
Composer is a dependency management tool for PHP that allows developers to declare and manage the external libraries and packages their projects depend on. It simplifies the process of installing, updating, and loading these dependencies into a PHP project.
Key Features and Functionalities of Composer include:
- Dependency Declaration: In a file called
composer.json
, developers can specify the required packages and their respective versions that their PHP project depends on. This file serves as the manifest for the project’s dependencies. - Package Installation: Composer can read the
composer.json
file and automatically download and install the required packages and their dependencies from the central repository known as Packagist. - Version Constraint Resolution: When specifying dependencies, developers can use version constraints to define which versions of packages are acceptable for their project. Composer will attempt to find the best combination of packages that satisfy all the version constraints.
- Autoloading: Composer generates an autoloader file, which automatically loads the classes from the installed packages, making it easy to use external libraries without manually including their files.
- Update Management: With Composer, you can update your project’s dependencies to newer versions or specific versions as required, while ensuring that the updated versions are compatible with each other.
- Integration with Frameworks: Many PHP frameworks, including Laravel, Symfony, and Yii, use Composer as their package manager, making it seamless to manage dependencies within those frameworks.
- Packagist Integration: Packagist (https://packagist.org/) is the primary package repository for PHP packages. It hosts a vast collection of libraries and packages that are available for use via Composer.
Using Composer simplifies the process of setting up and maintaining PHP projects by managing dependencies in a standardized and efficient way. It has become an essential tool in the PHP ecosystem, enabling developers to leverage the work of others and build robust applications with ease.
What is HTTP middleware?
HTTP middleware is a concept commonly used in web development, particularly in the context of web frameworks and applications that follow the Model-View-Controller (MVC) architectural pattern. Middleware acts as a bridge between the incoming HTTP request and the application’s response, allowing developers to perform certain actions and processing before the request reaches the application’s core logic or after the response is generated.
In the context of web frameworks like Laravel, Symfony, Express.js, or Django, middleware functions as a series of filters or layers that intercept and process incoming HTTP requests. Each middleware can perform specific tasks, such as authentication, logging, input validation, CORS handling, caching, or any other application-specific operation.
Here’s how HTTP middleware typically works in a request-response cycle:
- Incoming HTTP Request: When a client sends an HTTP request to the web application, it passes through a stack of middleware before reaching the application’s core logic (controllers or handlers).
- Middleware Processing: Each middleware in the stack can examine, modify, or handle the incoming request. For example, an authentication middleware might check if the user is logged in and authorized to access the requested resource. If not, it can redirect the user to a login page or return an error response.
- Application Core Logic: After passing through all the middleware, the request reaches the application’s core logic (controllers or handlers). This is where the primary business logic of the application is executed.
- Outgoing HTTP Response: Once the application generates a response, it goes through the stack of middleware again, but this time in the reverse order. Each middleware can modify the response or perform additional actions before it is sent back to the client.
Middleware provides a flexible and reusable way to handle cross-cutting concerns in web applications, such as authentication, security, logging, and more. It helps keep the application’s core logic clean and focused while allowing developers to modularize common functionality and apply it consistently across different routes and endpoints.
The specific implementation and usage of middleware may vary depending on the web framework being used, but the fundamental idea remains the same: intercepting and processing HTTP requests and responses through a chain of filters or middleware components.
Name aggregates methods of query builder
The query builder is a feature commonly found in modern web frameworks and database libraries that allows developers to construct SQL queries programmatically using a fluent interface. While the specific methods available may vary depending on the query builder implementation, I’ll provide a list of commonly used aggregate methods typically found in query builders:
count
: Used to retrieve the number of rows matching the query criteria.sum
: Calculates the sum of a specific column in the query result.avg
: Computes the average value of a column in the query result.min
: Finds the minimum value of a column in the query result.max
: Identifies the maximum value of a column in the query result.groupBy
: Groups the query result by one or more columns.having
: Applies conditions to grouped query results when used withgroupBy
.distinct
: Returns only distinct (unique) values from the query result.
These aggregate methods enable developers to perform various calculations and operations on data retrieved from the database efficiently and conveniently. They are particularly useful when dealing with large datasets and performing statistical operations on the data. It’s important to consult the specific documentation of the query builder or ORM (Object-Relational Mapping) library being used for the full list of available methods and their usage details.
What is a Route?
In web development, a route refers to the mapping between a URL (Uniform Resource Locator) and the corresponding handler or controller that processes the incoming HTTP request. Routes play a crucial role in web frameworks and applications as they determine how the application responds to different URLs.
When a user or client makes an HTTP request to a web server, the server needs to determine which part of the application should handle the request and generate the appropriate response. This is where routes come into play. They define the paths or URLs that users can access, and they map those URLs to specific actions or functions that will process the request and generate the response.
Routes are typically defined in a configuration file in the web framework or application. The configuration file specifies the URL patterns, HTTP methods (GET, POST, PUT, DELETE, etc.), and the corresponding controller or handler functions that should be executed when a request matches a particular route.
For example, in the context of a PHP web framework like Laravel or Symfony, a route might look like this:
Route::get('/users', 'UserController@index');
In this example, when a user accesses the URL /users
with an HTTP GET request, the index
method of the UserController
will be executed to handle the request and return the appropriate response, such as a list of users.
Routes allow developers to define a logical structure for their web applications and provide a clean separation between URLs and the underlying application logic. They make it easy to manage and organize different parts of the application and provide a user-friendly way for clients to interact with the server’s resources.
What do you mean by bundles?
In web development, the term “bundles” can have different meanings depending on the context. Here are two common interpretations:
- Bundles in Web Frameworks:
In some web frameworks, like Symfony (PHP) and Play Framework (Java/Scala), a bundle is a modular and self-contained unit of code that packages together related functionality, including controllers, templates, configuration files, and other resources. Bundles help organize code into reusable and decoupled components, making it easier to manage and maintain large web applications. Each bundle focuses on a specific set of features or functionalities, and they can be combined and reused across different projects.
For example, in Symfony, a bundle might encapsulate the user authentication system, while another bundle could handle blog functionality. This modular approach promotes code reusability and maintainability by separating concerns and keeping code related to specific features isolated.
- Bundles in Front-End Development:
In the context of front-end development, particularly when using tools like Webpack or Parcel, a bundle refers to the output of the build process, where multiple JavaScript, CSS, and other assets are combined into a single file (or a set of files) to reduce the number of HTTP requests required for loading a web page. Bundling assets can improve web page performance by reducing the overall file size and minimizing network latency.
When bundling front-end assets, developers typically include libraries, frameworks, and custom code into a single bundle or multiple bundles based on the application’s requirements. This bundling process is often part of the build pipeline to optimize the web application for production deployment.
In both interpretations, bundles aim to simplify the development process, enhance maintainability, and improve the performance of web applications. However, the specific implementation and usage of bundles may vary depending on the web framework or front-end build tools being used.
Explain important directories used in a common Laravel application
In a common Laravel application, there are several important directories that play specific roles in organizing and managing different parts of the project. Understanding these directories is essential for developing and maintaining a Laravel application. Here are the key directories used in a typical Laravel application:
app
: This directory is the heart of the application and contains the majority of the application’s code. It includes subdirectories for controllers, models, middleware, service providers, and more. Theapp
directory is where you’ll define the core business logic and functionality of your application.config
: This directory contains configuration files for various aspects of the application, such as database connections, application settings, services, and other third-party integrations. Laravel’s configuration files provide a convenient way to customize the behavior of the application.database
: This directory is used for database-related files, including migrations, seeds, and factories. Migrations allow you to version-control and manage changes to the database schema, while seeds and factories help generate test data for development and testing purposes.public
: This directory is the web server’s document root, and it contains theindex.php
file, which serves as the entry point for all incoming requests. Thepublic
directory also holds assets like CSS, JavaScript, and images that can be accessed directly by web clients.resources
: This directory contains all non-PHP resources used by the application, such as Blade templates, language files, and front-end assets. Theviews
subdirectory holds Blade templates used for rendering HTML pages.routes
: This directory contains route definition files that map URLs to controller actions. Laravel supports several types of routes, including web routes, API routes, and console routes.storage
: This directory is used to store files generated by the application, such as logs, cache files, and uploaded files. It includes subdirectories forapp
,framework
, andlogs
, among others.tests
: This directory contains test files for the application. Laravel encourages developers to write tests to ensure the stability and correctness of the codebase.vendor
: This directory is not created by developers but is automatically generated when dependencies are installed using Composer. It holds all the third-party libraries and packages required by the application.
These directories provide a well-organized structure for developing Laravel applications, and understanding their purposes can significantly streamline the development process and facilitate collaboration within a team.
Explain reverse routing in Laravel
Reverse routing, also known as named routing or route naming, is a feature in the Laravel framework that allows developers to generate URLs based on the defined routes. In other words, it allows you to create URLs dynamically without hardcoding them in your application code. This provides several benefits, such as maintaining consistency and flexibility when changing the structure of your application’s routes.
When you define a route in Laravel, you can give it a unique name using the name
method. For example:
Route::get('/users', 'UserController@index')->name('users.index');
In this example, we’ve given the route a name of 'users.index'
. Now, with reverse routing, you can generate the URL for this route using the route
helper function or the url
method:
// Using the route helper function
$url = route('users.index');
// Using the url method
$url = url('users');
The route
function takes the name of the route as its argument and returns the full URL for that route. The url
method can also be used to generate the URL directly by providing the route URI. Both methods will output the same URL for the named route:
http://example.com/users
The real power of reverse routing becomes apparent when you need to make changes to your routes. For instance, if you decide to change the URI of the /users
route to /members
, you only need to update the route definition:
Route::get('/members', 'UserController@index')->name('users.index');
Since the route has a name, all the places in your code where you generated the URL using that name will automatically be updated to use the new URI. This saves you from manually updating each occurrence of the URL throughout your application.
Reverse routing not only simplifies URL generation but also helps make your code more maintainable and less error-prone. It encourages the use of meaningful route names, making your codebase more readable and self-documenting.
Explain traits in Laravel
Traits in Laravel (and in PHP in general) are a mechanism that allows developers to reuse sets of methods in different classes without using traditional inheritance. Traits provide a way to horizontally share code among classes, enabling composition of behavior, which can be particularly useful when a class needs to have functionalities from multiple sources.
Here’s how traits work in Laravel:
- Defining a Trait:
A trait is defined as a PHP file containing a set of methods that you want to share across multiple classes. Traits do not have properties and cannot be instantiated directly. Instead, they are meant to be used as building blocks for other classes.
// Example of a trait definition
trait Loggable {
public function log($message) {
// Code to log the message
}
}
- Using a Trait in a Class:
To use a trait in a class, you simply use theuse
keyword followed by the trait’s name. The methods defined in the trait will become part of the class and can be used as if they were declared directly in the class.
class User {
use Loggable;
// Now the User class has access to the log method from the Loggable trait
public function someMethod() {
$this->log('This is a log message');
}
}
- Method Resolution Order:
If a class uses a trait and implements a method with the same name as a method in the trait, the method from the class takes precedence (method in the class overrides the trait’s method). This is known as “method resolution order.”
trait ExampleTrait {
public function sayHello() {
echo 'Hello from the trait!';
}
}
class MyClass {
use ExampleTrait;
public function sayHello() {
echo 'Hello from the class!';
}
}
$obj = new MyClass();
$obj->sayHello(); // Output: Hello from the class!
Traits provide a way to achieve code reuse without creating a complex class hierarchy through inheritance. They promote modularity, making it easier to organize and maintain code by separating concerns into smaller, manageable pieces. In Laravel, traits are often used to share common functionality across different classes, such as adding common methods to controllers, models, and other components of the application. However, it is essential to use traits judiciously and avoid excessive code sharing to maintain clarity and avoid conflicts.