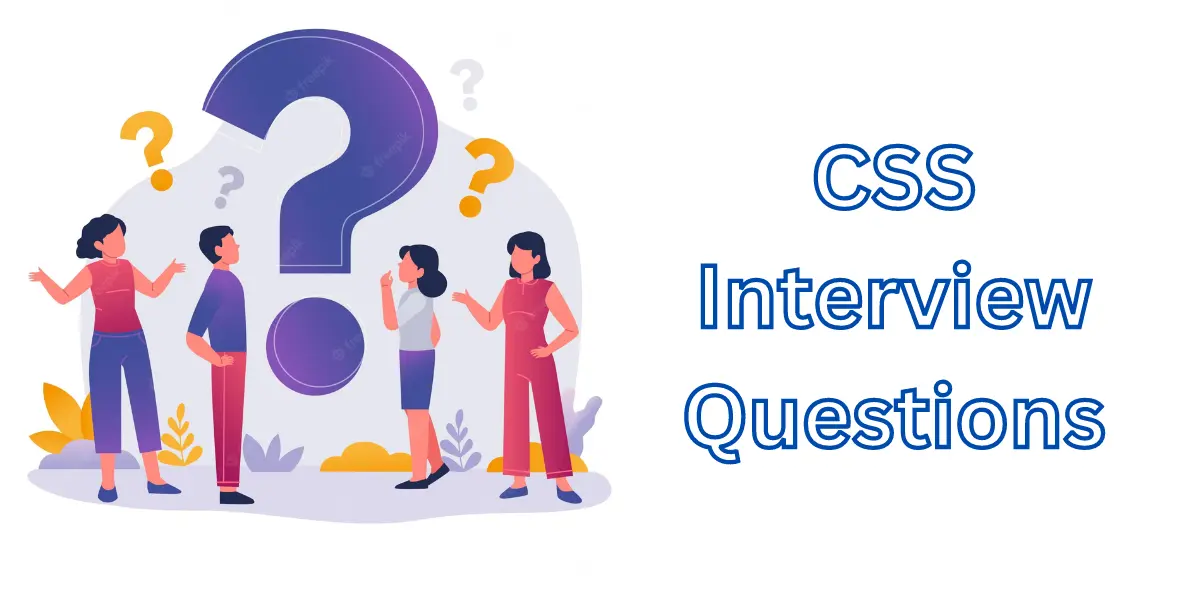
CSS Interview Questions
What is CSS?
CSS stands for Cascading Style Sheets. It is a style sheet language used to describe the presentation and layout of HTML (HyperText Markup Language) documents or other markup languages. CSS is an essential component of web development and is used to control the appearance and visual design of web pages.
The primary purpose of CSS is to separate the content and structure of a webpage from its presentation. By doing so, it allows web developers to define styles, such as colors, fonts, margins, padding, positioning, and more, independently from the HTML content. This separation of concerns makes it easier to maintain and update the design of a website, as changes to the CSS file can be applied globally to all pages using that stylesheet.
CSS operates on a “cascading” principle, where multiple CSS rules can apply to an element, and the final presentation is determined by a set of rules based on factors like specificity, inheritance, and order of appearance. This allows for flexible and modular design.
CSS can be used in several ways:
- Inline Styles: CSS styles can be applied directly to HTML elements using the
style
attribute. However, inline styles are generally not recommended due to limited reusability and maintainability.
<p style="color: blue; font-size: 16px;">This is a paragraph with inline styles.</p>
- Internal Stylesheet: CSS styles can be embedded within the
<style>
element in the head section of an HTML document. This method is suitable for applying styles to a specific page.
<!DOCTYPE html>
<html>
<head>
<title>Internal Stylesheet Example</title>
<style>
p {
color: blue;
font-size: 16px;
}
</style>
</head>
<body>
<p>This is a paragraph with internal styles.</p>
</body>
</html>
- External Stylesheet: CSS styles can be defined in an external CSS file and linked to the HTML document using the
<link>
element. This method is widely used and recommended, as it promotes better separation of concerns and reusability.
CSS file (styles.css):
p {
color: blue;
font-size: 16px;
}
HTML file:
<!DOCTYPE html>
<html>
<head>
<title>External Stylesheet Example</title>
<link rel="stylesheet" href="styles.css">
</head>
<body>
<p>This is a paragraph with external styles.</p>
</body>
</html>
CSS is a powerful tool for web designers and developers, allowing them to create visually appealing and responsive web pages that adapt to different devices and screen sizes. It is an integral part of modern web development and plays a significant role in creating engaging and user-friendly websites.
What are the advantages of using CSS?
Using CSS (Cascading Style Sheets) in web development offers numerous advantages that contribute to more efficient, consistent, and visually appealing web pages. Here are some of the key advantages of using CSS:
- Separation of Concerns: CSS allows for a clear separation of presentation and content. This means that you can define the layout, colors, and styles of your web pages separately from the HTML structure. This separation makes it easier to maintain and update the design without touching the underlying content.
- Consistency and Reusability: CSS enables you to create styles once and apply them to multiple elements or pages. By using external stylesheets, you can apply the same styles across an entire website, ensuring a consistent and uniform look and feel.
- Flexibility and Modularity: CSS supports cascading and inheritance, allowing you to create flexible and modular designs. Styles can be applied at different levels, making it easy to override or customize styles for specific elements without affecting the rest of the page.
- Page Load Speed: By using external stylesheets, you can cache CSS files on the client’s browser, reducing page load times for subsequent visits. This caching mechanism enhances the overall performance of the website.
- Improved SEO: Well-structured HTML with proper use of CSS can positively impact search engine optimization (SEO). Search engines prefer clean and organized code, which CSS facilitates by separating content from presentation.
- Accessibility: CSS allows you to create accessible web pages by providing semantic markup and improving the use of headings, lists, and other HTML elements. This helps screen readers and other assistive technologies interpret the content more effectively.
- Responsive Design: CSS plays a crucial role in creating responsive web design. By using media queries and flexible layout techniques, you can make your website adapt to different screen sizes and devices, such as desktops, tablets, and smartphones.
- Print Stylesheets: CSS allows you to define specific styles for printing, ensuring that the printed version of your web pages looks appropriate and professional.
- Maintainability: Centralizing styles in external CSS files makes it easier to update and maintain the design of your website. Changes made to the CSS affect all pages that link to that stylesheet.
- Global Control: CSS gives you the ability to control various aspects of your website’s appearance globally. If you need to change the color scheme or font styles, you can do so by modifying a few lines of CSS code.
Overall, CSS provides a powerful and efficient way to style web pages, improving the design, maintainability, and user experience of your website. Its flexibility and versatility have made it an essential tool for web designers and developers worldwide.
What are the limitations of CSS?
While CSS is a powerful and widely used tool for styling web pages, it also has some limitations and challenges. Understanding these limitations can help web developers make informed decisions and find appropriate solutions when designing and implementing their websites. Some of the main limitations of CSS are:
- Browser Compatibility: Different web browsers may interpret CSS rules slightly differently, leading to inconsistencies in how the page is displayed across different browsers. This often requires extra effort and testing to ensure cross-browser compatibility.
- Complex Layouts: Creating complex layouts, especially those involving multiple columns, equal-height columns, or advanced positioning, can be challenging in CSS. It may require workarounds or the use of CSS frameworks.
- Lack of Variables: Unlike some programming languages, CSS does not have built-in support for variables. This makes it difficult to reuse colors, fonts, or other styles consistently throughout the stylesheet. Preprocessors like Sass or LESS address this limitation.
- Limited Control over Elements’ Order: CSS provides limited control over the order in which elements are displayed. For complex layout scenarios, developers may need to use techniques like flexbox or CSS grid, which may not be fully supported in older browsers.
- Limited Mathematical Operations: CSS has limited support for mathematical operations. While it can handle basic calculations for properties like width and height, it lacks more complex mathematical capabilities.
- Print Styling Challenges: Creating consistent print styles can be challenging due to the variation in printing capabilities of different browsers and printer configurations.
- Page Break Control: CSS offers limited control over page breaks in printed documents, which may lead to unpredictable results when printing lengthy web pages.
- Performance Impact: Applying extensive and complex CSS styles to a page can impact performance, leading to slower page load times, especially on mobile devices with limited processing power.
- Lack of Complete Layout Control: CSS provides limited control over vertical alignment within a container and lack of “parent selector” support, making certain layout tasks more difficult to achieve.
- CSS Code Maintenance: As CSS files grow in size and complexity, code maintenance can become challenging, and it may be challenging to identify and fix specific styling issues.
Despite these limitations, CSS remains a critical tool for web design and presentation, and many of these challenges can be overcome with careful planning, the use of modern CSS techniques, and cross-browser testing. Additionally, CSS continues to evolve, with new features and improvements regularly introduced to address some of these limitations.
What are the different types of Selectors in CSS?
In CSS, selectors are patterns used to target and style HTML elements on a web page. They allow you to select specific elements or groups of elements to apply styles to. There are several types of selectors in CSS, each with its specific syntax and usage. Here are some of the most common types of selectors:
- Universal Selector (
*
): The universal selector selects all elements on the page. It is often used to apply styles globally or as a base reset.
* {
margin: 0;
padding: 0;
}
- Type Selector: The type selector selects all elements of a specific type. It targets elements based on their HTML tag name.
p {
font-size: 16px;
}
- Class Selector (
.classname
): The class selector selects elements with a specific class attribute value. It is prefixed with a period (.
) and targets elements with a matchingclass
attribute.
.button {
background-color: blue;
}
- ID Selector (
#idname
): The ID selector selects a single element with a specificid
attribute value. It is prefixed with a hash (#
).
#header {
font-weight: bold;
}
- Grouping Selector: The grouping selector allows you to apply styles to multiple selectors at once. Selectors are separated by commas.
h1, h2, h3 {
color: red;
}
- Descendant Selector: The descendant selector selects an element that is a descendant of another specific element.
ul li {
list-style-type: square;
}
- Child Selector: The child selector selects an element that is a direct child of another specific element.
ul > li {
font-weight: bold;
}
- Adjacent Sibling Selector: The adjacent sibling selector selects an element that immediately follows another specific element.
h1 + p {
margin-top: 10px;
}
- Attribute Selector: The attribute selector selects elements based on their attribute values.
input[type="text"] {
border: 1px solid black;
}
- Pseudo-class Selector: The pseudo-class selector selects elements based on their state or position, such as
:hover
,:focus
,:nth-child()
, etc.
a:hover {
color: orange;
}
These are just some of the common CSS selectors. CSS provides a rich set of selectors that offer great flexibility in targeting and styling specific elements on web pages. Understanding and using CSS selectors effectively is essential for writing efficient and maintainable CSS stylesheets.